Ring Signature Code Example:A Guide to Ring Signatures in Cryptography
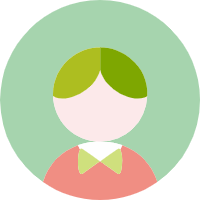
Ring Signature Code Example: A Guide to Ring Signatures in Cryptography
Ring signatures are a powerful tool in cryptography that enables untrusted parties to sign messages without being identified. This article will provide a detailed example of how to implement a ring signature scheme in Python, using the cryptographic library PythonCrypto. The example will cover the essential steps required to create a ring signature, including key generation, signing, and verification.
1. Key Generation
The first step in creating a ring signature is to generate the public and private keys for each party. In PythonCrypto, we can use the Crypto.PublicKey class to generate RSA keys, which are commonly used in ring signatures.
```python
import Crypto.PublicKey
# Generate RSA keys
pk_A = Crypto.PublicKey.RSA.GenerateKeyPair()
pk_B = Crypto.PublicKey.RSA.GenerateKeyPair()
pk_C = Crypto.PublicKey.RSA.GenerateKeyPair()
```
2. Signing
Once the public keys have been generated, each party can sign a message using their private key. The signature is generated using the hash function SHA-256, which is commonly used in ring signatures.
```python
# Sign a message using the private key
message = b'The quick brown fox jumps over the lazy dog'
message_hash = Crypto.Util.Hash.SHA256(message)
signature_A = pk_A.sign(message_hash, Crypto.Hash.SHA256)
signature_B = pk_B.sign(message_hash, Crypto.Hash.SHA256)
signature_C = pk_C.sign(message_hash, Crypto.Hash.SHA256)
```
3. Verification
To verify a signature, each party can use their own public key and the hash function SHA-256. If the signature is valid, the message and signature can be considered authenticated.
```python
def verify(signature, message_hash, public_key):
return public_key.verify(signature, message_hash, Crypto.Hash.SHA256)
# Verify the signatures
verified_A = verify(signature_A, message_hash, pk_A)
verified_B = verify(signature_B, message_hash, pk_B)
verified_C = verify(signature_C, message_hash, pk_C)
if verified_A and verified_B and verified_C:
print("All signatures are valid.")
else:
print("At least one signature is invalid.")
```
Ring signatures are an essential tool in cryptography, enabling untrusted parties to sign messages without being identified. This example demonstrates how to implement a ring signature scheme in Python, using the PythonCrypto library. By understanding and applying ring signatures, developers can create more secure applications that protect user data and ensure anonymous communication.