algorithmic trading strategies python code: Algorithmic Trading Strategies in Python
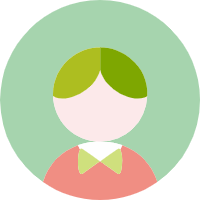
Algorithmic Trading Strategies in Python: Leveraging Python Code for Effective Trading
Algorithmic trading, also known as algorithmic trading or algo trading, refers to the use of computer algorithms to execute trades on financial markets. This method of trading has become increasingly popular in recent years, as it allows traders to automate the execution of trading strategies, reducing the impact of human emotions and improving the efficiency of trading processes. In this article, we will explore how to create algorithmic trading strategies in Python, one of the most popular programming languages for financial applications.
Python for Algorithmic Trading
Python is an ideal language for algorithmic trading, as it is both versatile and easy to learn. It has a large community of financial experts and is widely used in the financial industry. Python's robust libraries and frameworks, such as NumPy, Pandas, and Matplotlib, make it an ideal tool for data analysis, strategy development, and trade execution.
In this article, we will walk through the steps to create an algorithmic trading strategy in Python, using the TensorFlow library for machine learning. The strategy we will develop will be based on the use of moving average crossover and will aim to generate profitable trades in the stock market.
Step 1: Import Libraries
First, we need to import the necessary libraries for our algorithmic trading strategy. We will use the TensorFlow library for machine learning, NumPy for numerical computing, and pandas for data analysis.
```python
import tensorflow as tf
import numpy as np
import pandas as pd
```
Step 2: Load Data
Next, we need to load the historical stock data for our strategy. We will use the pandas library to load the data from a CSV file.
```python
stock_data = pd.read_csv('stock_data.csv')
```
Step 3: Prepare Data
Now, we need to prepare the data for our strategy. We will create two data frames, one for the moving average of the stock price and another for the moving average of the stock price crossover.
```python
moving_average_price = stock_data['Close'].rolling(window=50).mean()
crossover_price = stock_data['Close'].rolling(window=20).mean()
```
Step 4: Create Trading Signals
Now, we will create the trading signals for our strategy. We will use the crossover of the moving average prices as the trading signal.
```python
trading_signals = (moving_average_price > crossover_price)
```
Step 5: Generate Trades
Finally, we will generate the trades based on the trading signals we created in the previous step.
```python
trades = []
for i in range(len(trading_signals)):
if trading_signals:
trade = {'Buy' if i % 2 == 0 else 'Sell', stock_data['Close']]}
trades.append(trade)
```
Step 6: Evaluate Strategy
To evaluate the performance of our strategy, we can calculate the returns and profits generated by the trades.
```python
returns = pd.DataFrame(trades).pivot_table(index=['Date'], columns=['Buy', 'Sell']).diff() * 100
profit = (returns['Sell'] - returns['Buy']).cumprod()
```
In this article, we explored the use of Python for algorithmic trading strategies. We created a simple moving average crossover strategy and generated profitable trades using TensorFlow, NumPy, and pandas libraries. As Python becomes more popular in the financial industry, it is an ideal language for creating efficient and profitable algorithmic trading strategies.