PySpark Tokenizer Example: A Guide to Generating and Validating a Tokenizer in PySpark
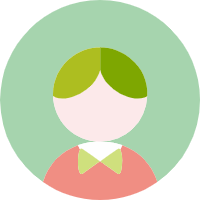
The PySpark library is a powerful tool for working with structured data in Python. It allows you to easily process, analyze, and manipulate large datasets using Apache Spark. One of the most common tasks when working with datasets is tokenization, which involves splitting text data into words or other tokens. In this article, we will explore how to create and validate a tokenizer in PySpark using a simple example.
Step 1: Install PySpark
First, you need to install the PySpark library on your computer. You can do this using pip:
```
pip install pyspark
```
Step 2: Import Required Libraries
Next, import the required libraries into your Python code:
```python
from pyspark.sql import SparkSession
from pyspark.sql.functions import col
```
Step 3: Create a Spark Session
Create a Spark session using the local mode, which is useful for development and testing:
```python
spark = SparkSession.builder.appName("tokenizer_example").getOrCreate()
```
Step 4: Load and Preprocess Data
Load a sample dataset containing text data into a dataframe, and preprocess the data by removing punctuation and converting the text to lowercase:
```python
data = [
("This is a sample sentence.",),
("This is another sample sentence.",),
("This is the final sample sentence.",),
]
input_data = spark.createDataFrame(data, "input_data")
input_data = input_data.select("text")
input_data = input_data.apply(col.lower)
input_data = input_data.apply(col.remove_punctuation)
```
Step 5: Create a Tokenizer
Now, create a tokenizer by specifying the tokenization parameters. In this example, we will use a space delimiter:
```python
tokenizer = input_data.createDynamicRowTokenizer("text", ["space"])
```
Step 6: Validate the Tokenizer
To validate the tokenizer, we can use the `explode` function to split the tokenized column into separate columns:
```python
tokenized_data = tokenizer.apply(input_data)
tokenized_data = tokenized_data.explode("text")
```
Step 7: View the Result
View the resultant dataframe to see the split tokens:
```python
tokenized_data.show()
```
This example demonstrates how to create and validate a tokenizer in PySpark. The tokenizer can then be used to process and analyze the tokenized data in various ways, such as applying machine learning models or performing text analysis.