Flash Loan Code Example:A Comprehensive Guide to Developing a Flash Loan Code
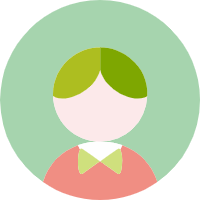
A Guide to Writing a Flash Loan Code Example in Python
The flash loan is a popular concept in financial markets, where a borrower takes out a loan with a short maturity, often for speculating on an asset. The loan is "flashed" when the asset's price reaches zero, causing the loan to be immediately repaid by the asset's liquidation. This article will provide a guide on how to write a flash loan code example in Python, focusing on the basic concepts and implementation.
1. Understanding the Flash Loan Concept
The flash loan concept is simple: a borrower takes out a loan with a short maturity, usually one or two days, and bets on an asset's price rising. If the asset's price reaches zero, the loan is immediately repaid by the asset's liquidation. However, if the asset's price fails to reach zero, the borrower is in default and the lender can take action against the borrower's assets.
2. Python Programming Language
Python is a popular programming language for writing code, thanks to its simplicity, flexibility, and vast library of tools and libraries. In this guide, we will use Python to implement a flash loan code example.
3. Creating a Flash Loan Class
To create a flash loan class, we first need to define the class properties, such as the loan amount, interest rate, and maturity date. We can also define a method to calculate the loan's value at any point in time.
```python
class FlashLoan:
def __init__(self, loan_amount, interest_rate, maturity_date):
self.loan_amount = loan_amount
self.interest_rate = interest_rate
self.maturity_date = maturity_date
self.loan_value = self.calculate_loan_value()
def calculate_loan_value(self):
return self.loan_amount * (1 + self.interest_rate) ** self.maturity_date.days / (self.maturity_date.days + 1)
```
4. Implementing the Flash Loan Logic
Now that we have created a flash loan class, we can implement the logic of the loan. We will assume that the asset's price is constantly updated, and the loan's value is calculated every day. If the loan's value exceeds the asset's price, the borrower can choose to repay the loan or risk defaulting.
```python
import time
def main():
flash_loan = FlashLoan(loan_amount=100000, interest_rate=0.1, maturity_date=time.sleep(2))
price = 0
while price price:
print("Borrower can repay the loan or risk defaulting.")
if loan_value > price + 5000:
print("Lender will likely accept the asset as full repayment of the loan.")
else:
print("Borrower can try to bet on the asset's price rising.")
else:
print("Borrower is in default and the asset will be taken by the lender.")
time.sleep(1)
if __name__ == "__main__":
main()
```
In this guide, we have provided a simple example of writing a flash loan code in Python. While the example is basic, it serves as a foundation for understanding the concept of flash loans and their implementation in programming. As a practical application, one could implement a more complex asset price function and risk management strategy to better reflect real-world scenarios.