Algorithmic trading strategy using Python: Developing an Algorithmic Trading Strategy with Python
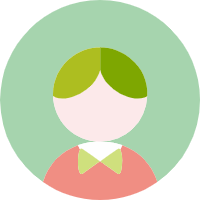
Algorithmic trading, also known as automated trading or algorithm-driven trading, has become an increasingly popular approach in the financial industry. It involves using computer programs to execute trades at high speeds and with minimal human intervention. Python, a highly versatile and widely used programming language, has emerged as a preferred tool for developing algorithmic trading strategies. In this article, we will explore the concept of algorithmic trading, its benefits, and how to create an algorithmic trading strategy using Python.
Benefits of Algorithmic Trading
The benefits of algorithmic trading include:
1. Improved execution efficiency: Algorithmic trading enables trades to be executed at high speeds, reducing the time taken to execute trades and minimizing the impact of market fluctuations.
2. Reduced trading costs: By automating the trading process, algorithmic trading can help reduce transaction costs associated with manual trading.
3. Improved trading accuracy: Algorithmic trading strategies can analyze large amounts of data and execute trades based on pre-defined criteria, leading to more accurate trade execution.
4. Reduced psychological factors: Human emotions can sometimes influence trading decisions, leading to suboptimal trade executions. Algorithmic trading can help remove these emotional factors and allow trades to be executed based on objective criteria.
5. Scalability: Algorithmic trading strategies can easily be scaled to accommodate larger trading volumes, making them suitable for large institutions and high-frequency traders.
Developing an Algorithmic Trading Strategy with Python
To develop an algorithmic trading strategy using Python, the first step is to select a suitable Python library for trading purposes. Some popular Python libraries for trading include Zipline, Pyfolio, and TA-Lib. In this article, we will use Zipline, a Python-based trading library that specializes in algorithmic trading.
The following steps will help you create an algorithmic trading strategy with Python:
1. Install the required libraries: Before starting the development process, make sure you have installed the required libraries, such as numpy, pandas, and zipline. You can install these libraries using pip, a Python package manager.
```
pip install numpy pandas zipline
```
2. Set up your trading account: Before developing an algorithmic trading strategy, you need to set up a trading account with a financial institution. This allows you to access real-time market data and execute trades using the strategy you develop.
3. Import the required libraries: In your Python code, import the required libraries and create a `trading_environment` object.
```python
import numpy as np
import pandas as pd
from zipline.api import run_effective_at
from zipline.api import SESSION_REALLY_LATE
```
4. Set up your trading strategy: Define your trading strategy by specifying the assets, trading rules, and portfolio constraints. You can use Zipline's built-in trading rules, such as SMA, BOLL, and MACD, or develop your own rules.
```python
def strategy_1(trading_environment):
signals = []
positions = []
# Your trading rules and signals here
return signals, positions
```
5. Run your trading strategy: Using the `run_effective_at` function, you can run your trading strategy at a specific time, such as the opening or closing of the market session.
```python
run_effective_at(SESSION_REALLY_LATE)
```
6. Execute trades: Once your trading strategy is set up, you can use the `execute_trade` function to execute trades based on your strategy's signals.
```python
trading_environment.execute_trade(
strategy_1,
trading_data.quotes.last(),
position_limit=0.01,
side=trading_environment.REASON_LONG)
```
7. Analyze your trading results: Use Zipline's reporting features to analyze your trading results, such as trades executed, profits, and losses.
Algorithmic trading strategy development using Python offers several benefits, including improved execution efficiency, reduced trading costs, and improved trading accuracy. By using Zipline, a popular Python-based trading library, you can create an algorithmic trading strategy with minimal effort and maximize your trading potential. As the technology and financial markets continue to evolve, the use of Python and other programming languages in algorithmic trading will likely become more prevalent, offering even more benefits for traders and investment firms.