mutable and immutable data types in python with example
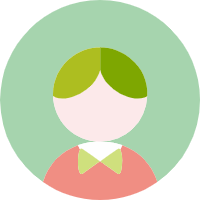
Mutable and Immutable Data Types in Python with Examples
Python is a highly versatile and versatile programming language, with a rich set of data types that can be used to represent and manipulate data. Data types in Python can be classified into two categories: mutable and immutable. Mutable data types can be modified after they are created, while immutable data types cannot. In this article, we will explore the different types of mutable and immutable data types in Python and provide some examples to help you better understand their usage.
Mutable Data Types in Python
1. List (ArrayList): A list is a mutable sequence data type that can contain any type of data. You can add, remove, or replace items in a list at any time.
```python
my_list = [1, 2, 3, 4]
my_list.append(5) # Add an item to the end of the list
print(my_list) # Output: [1, 2, 3, 4, 5]
```
2. Dictionary (HashMap): A dictionary is a mutable association data type that maps keys to values. You can add, remove, or replace keys and values in a dictionary at any time.
```python
my_dict = {'a': 1, 'b': 2, 'c': 3}
my_dict['d'] = 4 # Add a new key-value pair to the dictionary
print(my_dict) # Output: {'a': 1, 'b': 2, 'c': 3, 'd': 4}
```
3. Set (HashSet): A set is a mutable collection data type that contains unique elements. You can add, remove, or replace elements in a set at any time.
```python
my_set = {1, 2, 3, 4}
my_set.add(5) # Add an element to the set
print(my_set) # Output: {1, 2, 3, 4, 5}
```
Immutable Data Types in Python
1. String (String): A string is an immutable sequence data type that contains a sequence of characters. Once a string is created, its components cannot be modified.
```python
my_string = "Hello, World!"
# ...
print(my_string) # Output: "Hello, World!"
```
2. Tuple (Tuple): A tuple is an immutable sequence data type that contains a sequence of elements. Like strings, tuples cannot be modified after they are created.
```python
my_tuple = (1, 2, 3, 4)
# ...
print(my_tuple) # Output: (1, 2, 3, 4)
```
3. Number (Integer, Float, Complex): Values of numeric types (integers, floats, and complex numbers) are immutable once they are created.
```python
my_integer = 1
my_float = 2.5
my_complex = 3 + 4j
# ...
print(my_integer) # Output: 1
print(my_float) # Output: 2.5
print(my_complex) # Output: (3+4j)
```
Mutable and immutable data types in Python play a crucial role in the programming language's flexibility and efficiency. Understanding the differences between these types and their usage patterns can help you write more efficient and robust code. While immutable data types are generally preferred for performance reasons, their use also serves as a useful tool for avoiding data corruption and ensuring data consistency.