Immutable classes in Java:A Comprehensive Guide to Immutable Classes in Java
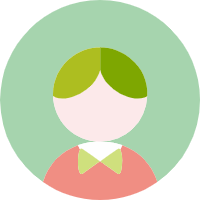
A Comprehensive Guide to Immutable Classes in Java
Immutable classes are a fundamental concept in the Java programming language. They represent objects that cannot be modified after creation, which provides several benefits, such as reducing the potential for confusion and error. In this article, we will explore the importance of immutable classes, their advantages, and how to create and use them effectively in Java.
Why Use Immutable Classes?
Immutable classes are beneficial for several reasons:
1. Memory efficiency: Since immutable objects cannot be modified after creation, they do not require additional memory for changes that may have occurred. This can lead to significant memory savings, especially when working with large datasets.
2. Simplified state management: By eliminating the need to manage state changes, immutable classes make it easier to reason about the code and minimize the risk of errors. This is particularly useful in multithreaded applications, where consistency and clarity are crucial.
3. Improved performance: Since immutable objects do not require additional memory, they can be passed around more efficiently, leading to faster execution. This is especially important in cases where multiple threads need to access the same objects simultaneously.
Creating Immutable Classes in Java
To create an immutable class in Java, you need to follow these steps:
1. Declare the class as static: Since immutable classes do not contain state, they cannot be instantiated. Instead, they can be accessed through their static methods and fields.
2. Declare all fields as final: Fields that are not modified after creation must be declared as final. This ensures that the values cannot be changed, even if access to the class is modified.
3. Provide constructors: Constructors are necessary to initialize the fields of the class. However, they cannot be used to modify the fields, as this would violate the immutable principle.
4. Implement static methods: These methods can be used to perform operations on immutable objects. They cannot modify the state of the class, as this would violate the immutable contract.
Example: Immutable Class in Java
Let's create an immutable class called "ImmutablePerson" to store information about a person:
```java
public class ImmutablePerson {
private final String name;
private final int age;
public ImmutablePerson(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public static ImmutablePerson of(String name, int age) {
return new ImmutablePerson(name, age);
}
}
```
In this example, the "ImmutablePerson" class is declared as static and all fields are declared as final. The constructor initializes the fields, and the "of" method is used to create new immutable objects.
Using Immutable Classes in Java
Once you have created immutable classes, you can use them in your applications as follows:
1. Create immutable objects: Use the "of" method to create new immutable objects with the required fields and values.
2. Access immutable objects: Access immutable objects through their static methods and fields. Do not attempt to modify the state of the object.
3. Concurrent access: When multiple threads need to access the same immutable objects, use synchronization to ensure consistency.
Immutable classes are an essential concept in Java programming, providing significant benefits such as memory efficiency, simplified state management, and improved performance. By creating and using immutable classes effectively, you can develop more reliable and efficient applications.